Suggestions
Use your own AI to help support agent
The suggestion API is in alpha state and signature will change before release.
Overview
The suggestion API allows you to integrate your own AI assistant into the Alcmeon processing page. An AI assistant allows a support agent to request suggestions to answer a conversation with a user. Then the agent can use or refuse the suggestion, and also modify the suggestion.
The suggestion API is asynchronous so that the AI assistant can take time to build the response.
To implement an AI assistant you need to:
- Implement the suggestion request webhook. When an agent asks for a suggestion, the question and the context is sent with a webhook_token. In the webhook, send back a 200 answer with a id as soon you receive the question, before starting to compute the answer.
- When the answer is generated, call the callback API with the answer, the id and the webhook_token of the question.
- Implement the suggestion statistics webhook to handle usage metrics. If the agent accepts or refuses the suggestion, and once the answer is sent to the user, a call to the statistics webhook is sent with the conversation, the proposed answer, if it was used, and the final answer (with the agent modifications).
How to configure your Alcméon platform
You need to connect with a administrator account on your Alcméon platform. You need to know which url connect to your server.
Get your instance_id
Your instance_id (or company_id) can be found in the Alcméon url: https://alcmeon.com/c/v3/#/{instance_id}/...
Create a ai.ssistant configuration
Go to setting, then ai.ssistant configuration, and create a new configuration.
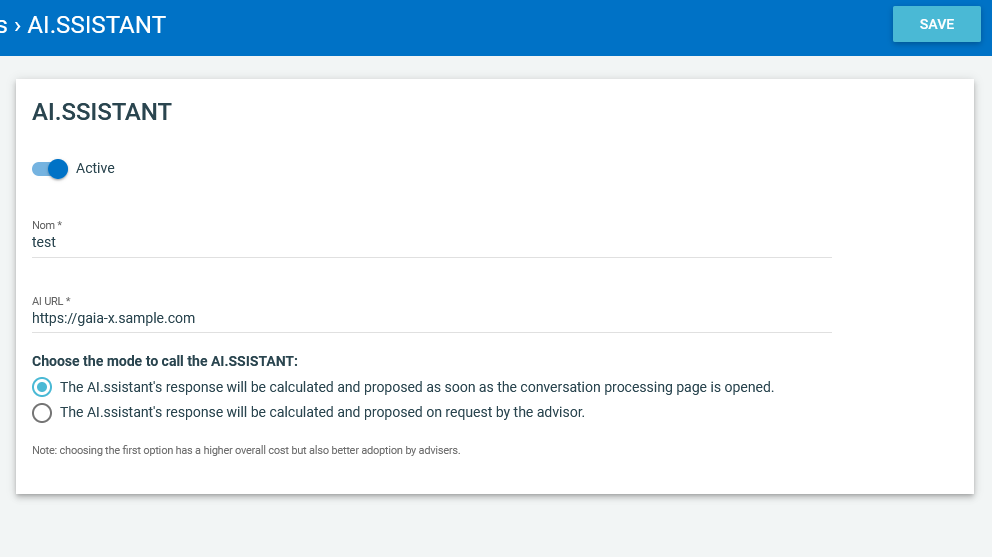
Choose a name, entre the url of your server, choose the mode, then save.
You will get a public key like this:
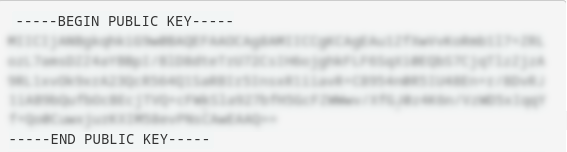
Copy this key and keep it safe.
Create an application
Go to Settings, then Applications.
Create an application with the permission AI Connector. You can give it any name you want.
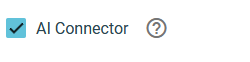
Then note the secret:
How to handle suggestion request
You must implement the suggestion request webhook.
The url used to invoke your webhook will be {server_url}/suggest-answer
Your webhook will receive the following parameters in the request body:
- version: Should be 1.0 by now
- question: The last message the user sent
- context: A list of context items (with a limit of 100 messages), each item has the following properties:
- role: user for a user message, bot for a bot message, adviser for a agent message, system for a system information
- date: Date of creation of the message
- content: The content of the message. For system informations, it's in the form key=value, with the following keys:
- adviser_lang : Language used by the agent
- adviser_user_id : Agent id
- adviser_user_name : Agent name
- user_id : User id
- user_external_id : External user id (depending on the channel)
- user_name : User name
- user_site_id : Channel id
- user_site_name : Channel name
- webhook_token: A token used to send back the suggestion once its computed
First you must answer with a id
(any string value) and a status
(should be started). The id is used to link the suggestion you will generate with the question, and must be send as suggestion_id
with the suggestion. The suggestion must be computed after the answer, and the suggestion will be send using the callback API.
This way Alcmeon platform will not have to wait for your AI to generate the suggestion.
How to protect your API
You can protect access to your webhooks by checking the header authorization.
To check the header authorization, extract the JWT that can be found after the 'Bearer ', decode it using the public key and a RS256 algorithm. You should then extract the company_id property and check its value.
Here is a sample python code using pyjwt to check an authorization header:
import jwt
# public_key should not be stored in code, but retrieve from environment variable or better yet from a secret manager
public_key = """-----BEGIN PUBLIC KEY-----
xxxxxxxxx
xxxxxxx
xxxx
-----END PUBLIC KEY-----"""
def authorization_error(authorization: str):
if authorization is None :
raise Exception("No authorization")
if not authorization.startswith('Bearer '):
raise Exception("Authorization not valid")
token = authorization[7:]
try:
data = jwt.decode(jwt=token, key=public_key, algorithms=["RS256"])
except jwt.exceptions.ExpiredSignatureError:
raise Exception("Expired token")
except Exception:
raise Exception("Invalid token")
token_company_id = data.get('company_id')
if token_company_id is None:
raise Exception("Invalid company_id")
How to send back suggestion
When you have a suggestion available, you need to send it using the callback API.
You will need to send suggestion_id\
and the webhook_token
so you should keep them.
Please note that this API is protected and you need to use authentication via HTTP Basic Auth with :
- username: your instance_id
- password: your application secret
Updated over 1 year ago